Quick example
By default, Angular Express kickstarts a modern Angular 1.x application that features:
- automatic compilation of ES6 with Babel
- pre-configured package management using jspm
- dynamic module loading using the SystemJS dynamic ES6 loader
- pre-configured unit testing with karma, mocha, chai and PhantomJS
- powerful built-in server based on Express 4 and Harp with preprocessor support for Jade, Markdown, EJS, Coffeescript, Sass, LESS and Stylus.
- compile to HTML, CSS & JavaScript and host it anywhere
First install the Angular Express CLI tool:
$ npm install -g ngx-cli
then use ngx init
to kickstart your new project:
# Create a project directory
$ mkdir new-project
$ cd new-project
# Initialize a new project
$ ngx init
# Install project dependencies
$ npm install
$ jspm install
The default boilerplate comes with powerful pre-configured development tools so you can start working on your application immediately:
# Start the development server that uses jspm and systemjs
# to dynamically load all scripts
$ npm run development-server
# Run unit tests
$ npm test
# Build all scripts to a minified bundle
$ npm build
# Start a production server that uses a minified bundle
# of the scripts
$ npm run production-server
# Compile a static version of your project to dist directory
$ npm run compile
Start the development server
From the root of your project, run:
# Start server in development mode
$ npm run development-server
and navigate your browser to http://localhost:9000
.
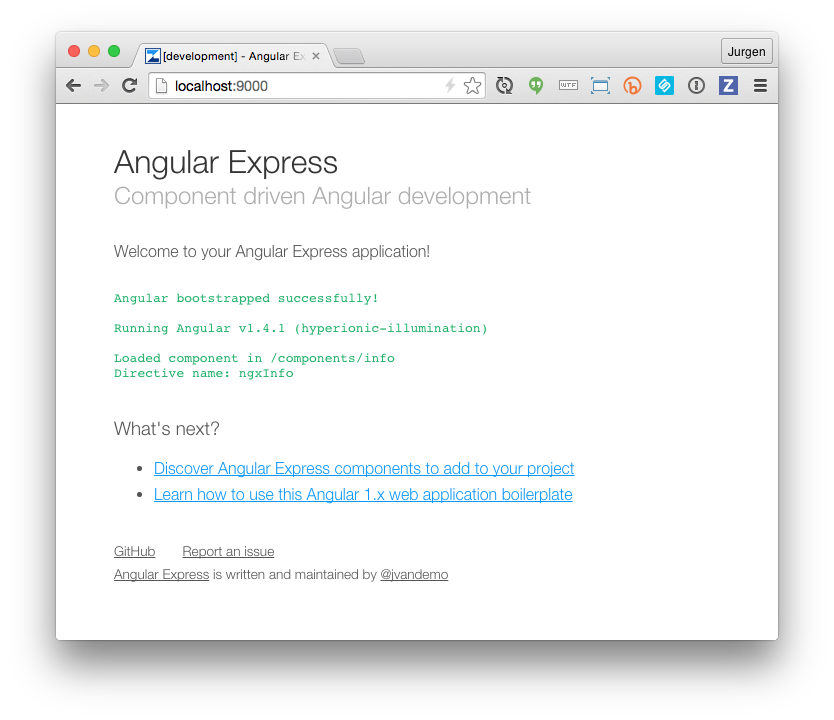
If you open up the console and refresh the page, you will see that jspm dynamically loads all the required scripts.
Sourcemaps are available to make debugging as convenient as possible.
Start the production server
From the root of your project, run:
# Build a minified script bundle
$ npm run build
# Start server in production mode
$ npm run production-server
and navigate your browser to http://localhost:9000
.
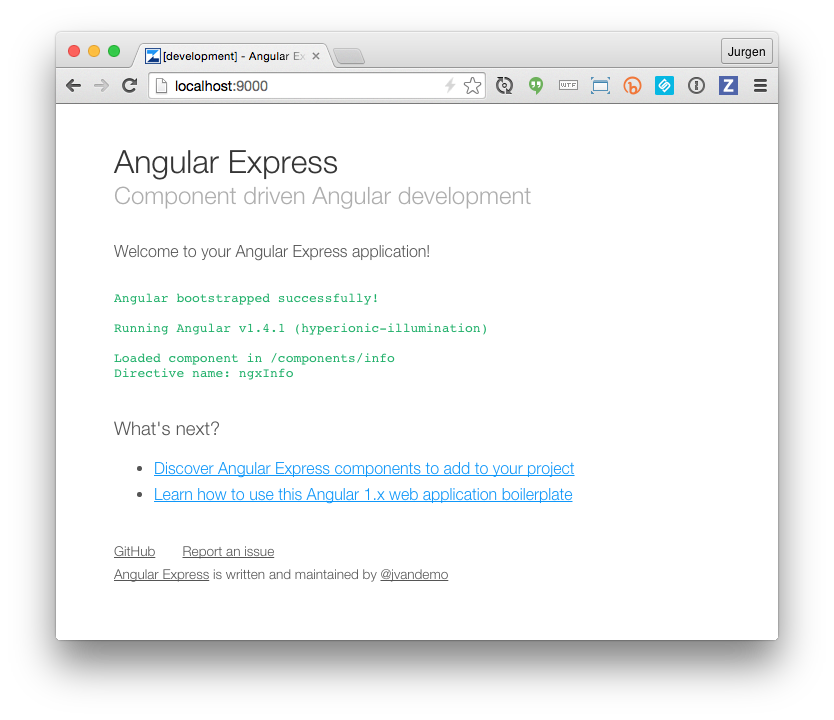
Now open up the console again and refresh the page. You will notice that all script requests have been replaced by one request to app/build.js
.
Compile your project to a static website
From the root of your project, run:
$ npm run compile
and a static version of your project will be generated in the dist
directory.
You can host this static version on any HTTP server.
If you use HTML5 mode in Angular, you will need to configure your server to rewrite URL's.
But wait, there is more!
After kickstarting your project you can easily add small Angular Express components on top of it:
# Add a component to handle ui-router state-not-found events
$ ngx install angular-ui-router-state-not-found-handler
# Add a component to handle ui-router state-change errors
$ ngx install angular-ui-router-state-change-error-handler
# Add a component to customize Bootstrap 3 using LESS
$ ngx install less-custom-bootstrap3
# Add some components that contain boilerplate to create ui-router states
$ ngx install angular-ui-router-component src/components/homepage
$ ngx install angular-ui-router-component src/components/about
$ ngx install angular-ui-router-component src/components/contact
$ ...
and import them in your Angular application as ES6 modules:
import angular from 'angular';
import errorHandler from 'components/angular-ui-router-state-change-error-handler/_build/index';
import homepage from 'components/hompage/_build/index';
import about from 'components/about/_build/index';
import contact from 'components/contact/_build/index';
/**************************************************************************
* Define Angular application
*************************************************************************/
var ngModule = angular.module('app', []);
ngModule.run(function () {
console.log('Angular bootstrapped!');
});
/**************************************************************************
* Initialize components and pass component specific options
*************************************************************************/
errorHandler(ngModule, { baseUrl: '/components/angular-ui-router-state-change-error-handler' });
homepage(ngModule, { baseUrl: '/components/homepage' });
about(ngModule, { baseUrl: '/components/about' });
contact(ngModule, { baseUrl: '/components/contact' });
to scaffold entire applications in just seconds!
Each component can accept component specific options making them highly reusable and flexible. In the example above, components are passed a baseUrl
to prepend to links in the view templates.
Once your application has been scaffolded, you can start adding your own code without having to worry about Angular Express.
Angular Express is not tied to your project or does not create hidden files in your repository. There are no dependencies either. It scaffolds the code for you and then backs off. No strings attached.
If, at any stage in your project, you want to add an additional existing component, you can run
ngx install
from the root of your project to install as many additional components as you like.
In short
Angular Express allows you to kickstart Angular applications by:
- initializing a boilerplate using
ngx init
- adding components to your application using
ngx install
Simple. Fast. Clean.
What's next?
Popular
Updated about 2 months ago